- Lab
- A Cloud Guru
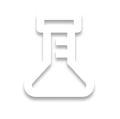
Overriding Comparison Operators in Python
When modeling systems in any programming language, we occasionally need our custom objects to be comparable. This is useful for when the class encapsulates a calculation based on some attributes and we want to sort them, or if we want to make direct comparisons to know if two instances are equivalent. In Python, we implement this sort of behavior by defining a suite of double underscore ("dunder") methods on our classes. These allow our custom class instances to be compared using the same operators that we would use if we wanted to know if one integer was greater than or equal to the next. In this hands-on lab, you'll be building a class to model a `Lead` for your sales team so that they can compare and rank leads in the system.
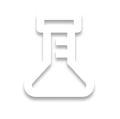
Path Info
Table of Contents
-
Challenge
Create the Lead Model and Calculate Lead Score
Before you can start working on the sorting of leads, you need to have a model. Your coworker has requested that you create the
Lead
class within thelead.py
file next totest_lead.py
. Be sure to create the initializer for the class and have it take the proper arguments:name
staff_size
estimated_revenue
effort_factor
With these implemented, you'll also want to add a method (or calculated property) to return the calculated lead score. Here's the formula to use:
1 / (staff_size / estimated_revenue * (10 ** (digits_in_revenue - digits_in_staff_size)) * effort_factor)
The automated tests assume that
lead_score
is a method, but you can adjust the test file if you'd rather it be calculated property instead.You can run the unit tests with the following command:
python -m unittest
-
Challenge
Implement the Equivalence Comparison Operators
The first set of operators that you need to implement are the
==
and!=
operators. These should compare the lead score of the twoLead
instances being compared. You can implement these using the__eq__
and__ne__
methods, and these methods should compare the lead scores of the instances. Implementing these methods should make a few more automated tests pass.You can run the unit tests with the following command:
python -m unittest
-
Challenge
Implement the Ordering Operators
With the equivalence operators implemented, you're ready to move onto implementing the ordering operators:
<
,>
,<=
, and>=
. These operators can be implemented by defining the__lt__
,__gt__
,__le__
, and__ge__
methods, and these methods should compare the lead scores of the instances. Implementing these methods should get the remaining automated tests to pass.You can run the unit tests with the following command:
python -m unittest
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.
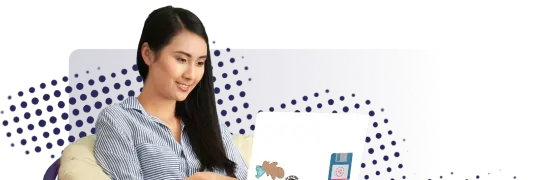
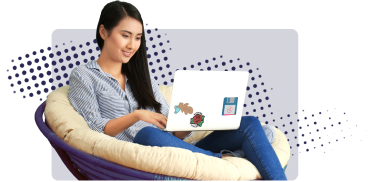