- Lab
- A Cloud Guru
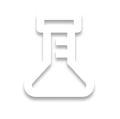
Processing Collections in Python Using Lambdas
Being able to perform actions on a collection is incredibly useful in any type of programming, and it is pretty common to need to perform a single action on each item. We could do this by using a loop, but there are built-in collection functions that can take a collection and a function or lambda to run each item through. In this hands-on lab, we utilize higher-order functions to process some existing lists by using lambdas. To feel comfortable completing this lab you'll want to know how to do the following: - Define and use lambdas. Watch "Defining and Using Lambdas" from the Certified Associate in Python Programming Certification course. - Use higher-order functions and collection functions. Watch "Using Collection Functions" from the Certified Associate in Python Programming Certification course.
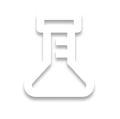
Path Info
Table of Contents
-
Challenge
Create the `sorted_by_name` List by Sorting the `people` List of Dictionaries
To sort our list of dictionaries we're going to use the
sorted
function. Not all of the values for thename
key in our dictionaries start with a capital letter, and because of that, we're going to want to convert all of the strings to lowercase before making the comparison. Thankfully, we can do this by passing a lambda for thekey
parameter. Here's how we can complete the first task:collection_processing.py
# 1) Sort the `people` list of dictionaries alphabetically based on the # 'name' key from each dictionary using the `sorted` function and store # the new list as `sorted_by_name` people = [ {"name": "Kevin Bacon", "age": 61}, {"name": "Fred Ward", "age": 77}, {"name": "finn Carter", "age": 59}, {"name": "Ariana Richards", "age": 40}, {"name": "Victor Wong", "age": 74}, ] sorted_by_name = sorted(people, key=lambda d: d['name'].lower()) assert sorted_by_name == [ {"name": "Ariana Richards", "age": 40}, {"name": "finn Carter", "age": 59}, {"name": "Fred Ward", "age": 77}, {"name": "Kevin Bacon", "age": 61}, {"name": "Victor Wong", "age": 74}, ], f"Expected sorted_by_name to equal '{sorted_by_name}' to equal '{[{'name': 'Ariana Richards', 'age': 40}, {'name': 'finn Carter', 'age': 59}, {'name': 'Fred Ward', 'age': 77}, {'name': 'Kevin Bacon', 'age': 61}, {'name': 'Victor Wong', 'age': 74}]}''"
Running the script, we should see the following error. This indicates that we've successfully met the requirements for the first task.
$ python3.7 collection_processing.py Traceback (most recent call last): File "collection_processing.py", line 36, in <module> ], f"Expected name_declarations to equal '{name_declarations}' to equal '{['Ariana Richards is 40 years old', 'finn Carter is 59 years old', 'Fred Ward is 77 y ears old', 'Kevin Bacon is 61 years old', 'Victor Wong is 74 years old']}'" AssertionError: Expected name_declarations to equal 'None' to equal '['Ariana Richards is 40 years old', 'finn Carter is 59 years old', 'Fred Ward is 77 years old', 'Kevin Bacon is 61 years old', 'Victor Wong is 74 years old']'
-
Challenge
Create the `name_declarations` List by Mapping over `sorted_by_name`
Our second task is to create a list where each string uses the
name
andage
parameters to build strings of<NAME> is <AGE> years old.
Let's create thename_declarations
variable:collection_processing.py
# 2) Use the `map` function to iterate over `sorted_by_name` to generate a # new list called `name_declarations` where each value is a string with # `<NAME> is <AGE> years old.` where the `<NAME>` and `<AGE>` values are from # the dictionaries. name_declarations = list( map(lambda d: f"{d['name']} is {d['age']} years old", sorted_by_name) ) assert name_declarations == [ "Ariana Richards is 40 years old", "finn Carter is 59 years old", "Fred Ward is 77 years old", "Kevin Bacon is 61 years old", "Victor Wong is 74 years old", ], f"Expected name_declarations to equal '{name_declarations}' to equal '{['Ariana Richards is 40 years old', 'finn Carter is 59 years old', 'Fred Ward is 77 years old', 'Kevin Bacon is 61 years old', 'Victor Wong is 74 years old']}'"
Note that we need to convert the result of
map
to be a list, otherwise ourname_declarations
variable will be amap
object.Running our script again, this is what we should see:
python3.7 collection_processing.py Traceback (most recent call last): File "collection_processing.py", line 50, in <module> ], f"Expected under_seventy to equal '{under_seventy}' to equal '{[{'name': 'Ariana Richards', 'age': 40}, {'name': 'finn Carter', 'age': 59}, {'name': 'Kevin Bacon', 'age': 61}]}'" AssertionError: Expected under_seventy to equal 'None' to equal '[{'name': 'Ariana Richards', 'age': 40}, {'name': 'finn Carter', 'age': 59}, {'name': 'Kevin Bacon', 'age': 61}]'
-
Challenge
Create the `under_seventy` List by Filtering and Sorting on the `sorted_by_name` List
Our last task is to filter the values in
sorted_by_name
so our output list only includes dictionaries that have anage
value less than 70. To achieve this, we're going to combinefilter
andsorted
to create our final list.collection_processing.py
# 3) Combine the `filter` and `sorted` functions to iterate over `sorted_by_name` to generate a # new list called `under_seventy` that only contains the dictionaries where the # 'age' key is less than 70, sorting the list by age. under_seventy = sorted( filter(lambda d: d['age'] < 70, sorted_by_name), key=lambda d: d['age'] ) assert under_seventy == [ {"name": "Ariana Richards", "age": 40}, {"name": "finn Carter", "age": 59}, {"name": "Kevin Bacon", "age": 61}, ], f"Expected under_seventy to equal '{under_seventy}' to equal '{[{'name': 'Ariana Richards', 'age': 40}, {'name': 'finn Carter', 'age': 59}, {'name': 'Kevin Bacon', 'age': 61}]}'"
Running our script one last time we should see no output because all of the assertions were true.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.
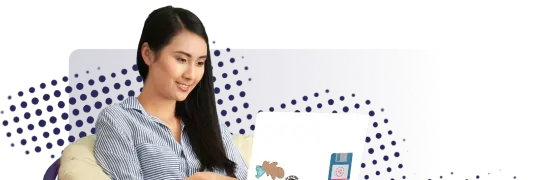
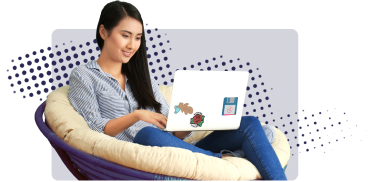