- Lab
- A Cloud Guru
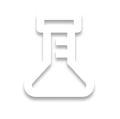
Using Composition in Python to Separate Concerns
Composition is an object-oriented programming technique that allows us to create flexible programs by separating the responsibilities across multiple classes that know how to interact with one another. This type of flexibility makes it easier to maintain and develop applications over long periods. In this hands-on lab, you'll be building a quiz system that utilizes some pre-written question classes and also requires you to create a new type of question without it changing how the overall quiz functions. Completing this lab will demonstrate that you know how to utilize composition when architecting a non-trivial program.
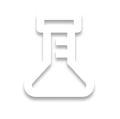
Path Info
Table of Contents
-
Challenge
Implement the Quiz Class with Support for Pre-Built Question Types
Create a file named
quiz.py
that contains the implementation for theQuiz
class.The class must have the following attributes:
questions
: A list of questions to display to the user.passing_percent
: The percentage of questions that must be correct to pass the quiz.actual_score
: The number of questions that were answered correctly.
The
Quiz
class is responsible for doing the following:- Randomly displaying questions one at a time to the user by using the
start
method. While a question is presented, the application should wait for user input and set the resulting message as the answer for the question before moving to the next question. - Calculating the number of total correct answers using the
score
method. This should set theactual_score
attribute and return the value. - Returning whether or not the quiz was passed using the
grade
method. The return value should be a tuple with the type of(float, bool)
, the actual percentage of questions that were answered correctly, and the pass/fail status.
Run the automated tests for the
Quiz
class using the following command:$ python -m unittest test_quiz.TestQuiz .... ---------------------------------------------------------------------- Ran 4 tests in 0.000s OK
-
Challenge
Implement the EssayQuestion Class
The
EssayQuestion
class needs to implement the same methods as theQuestion
,TrueFalseQuestion
, andMultipleSelectionQuestion
classes, but it will be simpler and shouldn't be a subclass ofQuestion
.Create a file named
essay_question.py
that contains the implementation for theEssayQuestion
class.The class must have the following attributes:
question_text
: The question itself.answer
: The user's response.answer_is_sufficient
: Whether the answer correctly answers the question.
The class is responsible for doing the following:
- Printing to the screen: When cast to a
str
, the question should return thequestion_text
. - Setting the answer: The
select
method should take an argument, ensure that it is a string, and set it as the value foranswer
. - Prompt for manual grading : The
grade
method should print thequestion_text
and the providedanswer
while prompting the grader with the question"Is this answer sufficient? [Yn]: "
(or something similar). The response should be read and thenanswer_is_sufficient
should be set to the boolean value before also returning that boolean value from the function.
There is a single test in the
test_quiz.py
file that utilizes this class, so once all of the tests are passing, you can feel confident that you've implemented the class correctly.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.
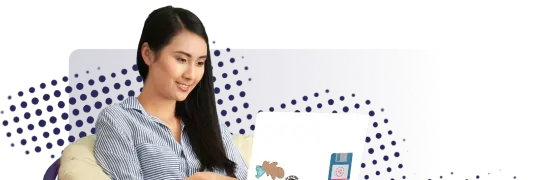
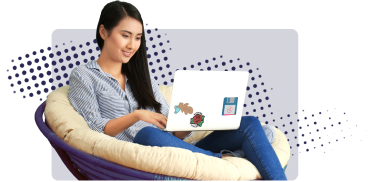