- Lab
- A Cloud Guru
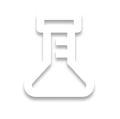
Using Python String Methods
Strings are the primary way that we interact with non-numerical data in programming, and the `str` type in Python provides us with a lot of powerful methods to make working with string data easier. In this hands-on lab, we'll be creating a script that can take a user-provided message and perform various actions on it before printing out those new results. To feel comfortable completing this lab you'll want to know how to do the following: * Utilize methods on the `str` class. Watch the "Using String Methods" from the Certified Entry-Level Python Programmer Certification course. * Working with list literals. Watch the "Lists" video from the Certified Entry-Level Python Programmer Certification course. * Using List functions and methods. Watch the "List Functions and Methods" video from the Certified Entry-Level Python Programmer Certification course.
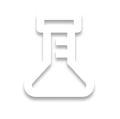
Path Info
Table of Contents
-
Challenge
Create the variations.py Script, Make It Executable with python3.7, and Accept User Input
For
variations.py
, we're going to place it in our home directory (~
) and we want to make sure that we can run it directly. To keep from being completely tied to the path of ourpython3.7
binary, we want to set up our shebang properly.Let's create the file and set the shebang:
~/variations.py
#!/usr/bin/env python3.7 # Python implementation here
With the file created, we need to also make sure that it's executable and we can do this using
chmod
:$ chmod u+x ~/variations.py
Next, we'll prompt the user for a message and store it off in a variable.
~/variations.py
#!/usr/bin/env python3.7 message = input("Enter a message: ")
Now if we run the script (
./variations.py
) it should prompt us for a message, and then exit without any errors. -
Challenge
Print the Lowercase, Uppercase, Title Case, and Capitalized Versions of the User's Input
Now that we have the
message
variable, we're going to print a few different things to the screen:- The lowercase version using
str.lower
- The uppercase version using
str.upper
- The title case version using
str.title
- The capitalized version using
str.capitalize
Let's use each of these methods combined with the
print
function:~/variations.py
#!/usr/bin/env python3.7 message = input("Enter a message: ") print("Lowercase:", message.lower()) print("Uppercase:", message.upper()) print("Capitalized:", message.capitalize()) print("Title Case:", message.title())
Now we can run the script to make sure that what we've written up to this point is working properly:
$ ./variations.py Enter a message: This Is My Message Lowercase: this is my message Uppercase: THIS IS MY MESSAGE Capitalized: This is my message Title Case: This Is My Message
- The lowercase version using
-
Challenge
Separate the String and Present the Individual Words as a List
For the remaining requirements of our script, we need to work with the individual words. Because of this, we're going to store the words off as a new variable,
words
. We can get the words by using thestr.split
method. After we've separated the message into words, let's also print them out:~/variations.py
#!/usr/bin/env python3.7 message = input("Enter a message: ") print("Lowercase:", message.lower()) print("Uppercase:", message.upper()) print("Capitalized:", message.capitalize()) print("Title Case:", message.title()) words = message.split() print("Words:", words)
Here's the script running so far:
$ ./variations.py Enter a message: This is a test Message! Lowercase: this is a test message! Uppercase: THIS IS A TEST MESSAGE! Capitalized: This is a test message! Title Case: This Is A Test Message! Words: ['This', 'is', 'a','test','Message!']
-
Challenge
Print the Alphabetic First and Last Words from the User's Input
We're going to sort the words in the
words
list alphabetically and save the new list tosorted_words
by using thesorted
built-in function. Lastly, we'll print the alphabetic first and last words. Let's do this by indexing to0
and-1
.~/variations.py
#!/usr/bin/env python3.7 message = input("Enter a message: ") print("Lowercase:", message.lower()) print("Uppercase:", message.upper()) print("Capitalized:", message.capitalize()) print("Title Case:", message.title()) words = message.split() print("Words:", words) sorted_words = sorted(words) print("Alphabetic First Word:", sorted_words[0]) print("Alphabetic Last Word:", sorted_words[-1])
Here's the final script running:
$ ./variations.py Enter a message: This is a test message! Lowercase: this is a test message! Uppercase: THIS IS A TEST MESSAGE! Capitalized: This is a test message! Title Case: This Is A Test Message! Words: ['This', 'is', 'a','test','message!'] Alphabetic First Word: This Alphabetic Last Word: test
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.
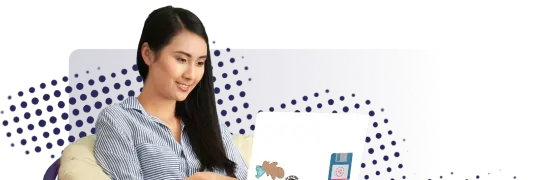
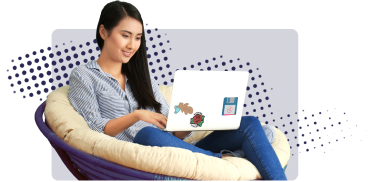