- Lab
- A Cloud Guru
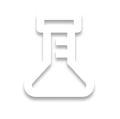
Using Python's os Module
Environment variables provide a great way to share information into an application, and being able to read environment variables is a key skill for programming. You will need basic Python programming and datetime skills for this lab: - [Certified Associate in Python Programming Certification](https://linuxacademy.com/cp/modules/view/id/470) - [Python's os module](https://docs.python.org/3/library/os.html#)
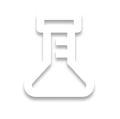
Path Info
Table of Contents
-
Challenge
Write Test
Using the best practice, Test-Driven Development, first write a test that will check to make sure your result matches what is wanted. There is a
# test
section for your code. Thetest_printer_config.ini
file contains the result you should check against. Rememberstreams
andfile i/o
to read the resulting file, the test file, and assert they are equal.After you have written the test, run
python write_config.py
.This should result in an
AssertionError
which you will fix in the next step.import configparser import os # data to be used data_list = [ ["author", "title", "pages", "due_date", "genre", "isbn"], ["Thompson, Keith", "Oh Python! My Python!", 1200, "2020-11-15", "biography", "000-1-000000-00-1"], ["Fritts, Larry", "Fun with Django", 150, "2020-06-23", "satire", "00-2-000000-00-2"] ] novel_text = "Numquam labore labore tempora. Dolore dolorem quisquam aliquam eius dolor non ipsum. Dolorem est velit tempora quaerat. Est magnam porro voluptatem dolore. Adipisci voluptatem consectetur dolore voluptatem voluptatem neque. Adipisci non modi quiquia etincidunt quisquam. Etincidunt consectetur dolore ut. Quaerat ut adipisci sit aliquam quisquam ut. Voluptatem labore porro porro.\n\nPorro modi numquam non sed dolor. Consectetur dolor adipisci quaerat aliquam adipisci. Etincidunt porro modi dolor neque numquam magnam neque. Ipsum consectetur ut eius ut. Dolor ut modi non est tempora labore sit.\n\nEtincidunt voluptatem quaerat consectetur sed est etincidunt ipsum. Ut adipisci numquam etincidunt porro porro. Dolore eius modi aliquam. Velit amet sed quaerat etincidunt est. Ut consectetur modi ipsum sed aliquam. Amet tempora quiquia eius voluptatem aliquam dolore ut. Etincidunt dolor non voluptatem eius sed non adipisci." # instantiate the configparser object config = configparser.ConfigParser() # add default section config["DEFAULT"] = { "pub_house": "Atlantic Publishing", "number_of_books": '2' } # add first book config["book1"] = { "author": data_list[1][0], "title": data_list[1][1], "genre": data_list[1][4], "text": novel_text } # add second book config["book2"] = { "author": data_list[2][0], "title": data_list[2][1], "genre": data_list[2][4], "text": novel_text } # print to file with open("printer_config.ini", "w") as f: config.write(f) # test # student input here with open("printer_config.ini", "r") as f: config_text = f.read() with open("test_printer_config.ini", "r") as f: expected_text = f.read() assert config_text == expected_text, f"Expected: {expected_text}\n\nGot: {config_text}"
-
Challenge
Change the File So It Has Correct Information
The
DEFAULT
section ofprinter_config.ini
should look like this:[DEFAULT] pub_house = Atlantic Publishing pub_house_address = 321 Publishers Way, Fargo, ND pub_house_phone = 555-555-5555
The environment variables are:
- PUB_HOUSE = "Atlantic Publishing"
- PUB_HOUSE_ADDRESS = "321 Publishers Way, Fargo, ND"
- PUB_HOUSE_PHONE = "555-555-5555"
Change the code in
write_config.py
to update thedefault
section.import configparser import os # data to be used data_list = [ ["author", "title", "pages", "due_date", "genre", "isbn"], ["Thompson, Keith", "Oh Python! My Python!", 1200, "2020-11-15", "biography", "000-1-000000-00-1"], ["Fritts, Larry", "Fun with Django", 150, "2020-06-23", "satire", "00-2-000000-00-2"] ] novel_text = "Numquam labore labore tempora. Dolore dolorem quisquam aliquam eius dolor non ipsum. Dolorem est velit tempora quaerat. Est magnam porro voluptatem dolore. Adipisci voluptatem consectetur dolore voluptatem voluptatem neque. Adipisci non modi quiquia etincidunt quisquam. Etincidunt consectetur dolore ut. Quaerat ut adipisci sit aliquam quisquam ut. Voluptatem labore porro porro.\n\nPorro modi numquam non sed dolor. Consectetur dolor adipisci quaerat aliquam adipisci. Etincidunt porro modi dolor neque numquam magnam neque. Ipsum consectetur ut eius ut. Dolor ut modi non est tempora labore sit.\n\nEtincidunt voluptatem quaerat consectetur sed est etincidunt ipsum. Ut adipisci numquam etincidunt porro porro. Dolore eius modi aliquam. Velit amet sed quaerat etincidunt est. Ut consectetur modi ipsum sed aliquam. Amet tempora quiquia eius voluptatem aliquam dolore ut. Etincidunt dolor non voluptatem eius sed non adipisci." # instantiate the configparser object config = configparser.ConfigParser() # add default section config["DEFAULT"] = { "pub_house": os.getenv("PUB_HOUSE"), "pub_house_address": os.getenv("PUB_HOUSE_ADDRESS"), "pub_house_phone": os.getenv("PUB_HOUSE_PHONE") } # add first book config["book1"] = { "author": data_list[1][0], "title": data_list[1][1], "genre": data_list[1][4], "text": novel_text } # add second book config["book2"] = { "author": data_list[2][0], "title": data_list[2][1], "genre": data_list[2][4], "text": novel_text } # print to file with open("printer_config.ini", "w") as f: config.write(f) # test with open("printer_config.ini", "r") as f: config_text = f.read() with open("test_printer_config.ini", "r") as f: expected_text = f.read() assert config_text == expected_text
Run
python write_config.py
.Congrats! You have successfully accessed environment variables using Python's
os
module.
What's a lab?
Hands-on Labs are real environments created by industry experts to help you learn. These environments help you gain knowledge and experience, practice without compromising your system, test without risk, destroy without fear, and let you learn from your mistakes. Hands-on Labs: practice your skills before delivering in the real world.
Provided environment for hands-on practice
We will provide the credentials and environment necessary for you to practice right within your browser.
Guided walkthrough
Follow along with the author’s guided walkthrough and build something new in your provided environment!
Did you know?
On average, you retain 75% more of your learning if you get time for practice.
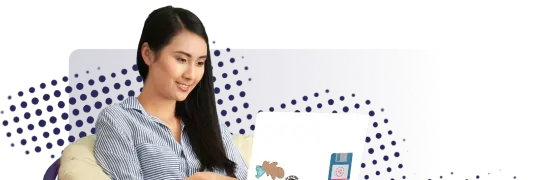
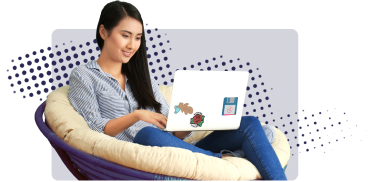